12Ghosts.com

English
Deutsch
BackGhosts
2ndBackup
ProfileCopy
Save Layout
Timer
Just Do It
JumpReg
SetTextColor
ShellX
Shutdown
Make it Easy
DeskTOP
Quick Menu
ShowTime
WinBzzzz
Download 900KB
Register
@ E-Mail
49 Ideas of Silence
Learn Typewriting
YourWeb.com
Link Collection
700 ACRONYMS
Windows NT
Visual C Tricks
PGP & Outlook
Outlook & VBA
My Dream PC
Excel 4.0 Macros
Homepage
Editorials
Ideas
Contact PACT
Register
@ E-Mail
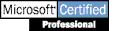
MCSE MCSD MCSI MCT


PACT supports
• Walker's Watchguard
• Citizens for Cycles
|
All information provided here are subject to change at anytime
without notice. No warranties, no liability, no guaranty for fitness of code.
Timer Homepage
FAQ
Frequently Asked Questions
Options
and Registry Values
Timer Options
There is just one command line option, /s
for silent mode. This causes the Timer to not
show the timer dialog after start. This is used in the startup shortcut
automatically.
Timer Registry Values
The values should be self-descriptive, however, here is the description of
all values.
ExeDescription |
Up to 100 characters. |
ExePath |
Up to 255 characters. |
ExeParameters |
Up to 255 characters. |
ExeWorkDir |
Up to 255 characters. |
StartNextDate |
'yyyy/MM/dd HH:mm:ss', 24-hour format, leading zero. |
StartEverySec |
recurring time in seconds, 0 = not recurring (if StartNextDate empty then after
logon takes precedence). |
StartSecAfterBoot |
If not 0, this value will be processed, takes precedence. (boot = launch of
Timer = after logon if in Startup). |
StartActivated |
1 = activated, else deactivated. |
StartDeleteAfter |
1 = delete after starting once, else only deactivate. |
StartWorkingOnly |
1 = recurring on working days only, that is, Monday through Friday, else run
every day. |
StartBell |
1 = play sound on action, else no sound. |
StartNotAfter |
'yyyy/MM/dd HH:mm:ss', 24-hour format, leading zero. |
WindowState |
0 Normal, 1 Min, 2 Max, 3 No Activate, 4 Min No Activate, 5 Hidden. |
PriorityLevel |
0 Realtime, 1 High, 2 Normal, 3 Idle. |
Example Registry File
Here's an example Registry file you can simply double-click to import it
into the registry. Copy the following lines and paste them into a new text document. Save
it with the extension *.reg.
REGEDIT4
[HKEY_CURRENT_USER\Software\PACT Software\Timer\myNewTimer]
"ExeDescription"="This is an example reminder"
"ExePath"=""
"ExeParameters"=""
"ExeWorkDir"=""
"StartNextDate"="1998/03/12 10:20:00"
"StartEverySec"=dword:00000000
"StartSecAfterBoot"=dword:00000000
"StartActivated"=dword:00000001
"StartDeleteAfter"=dword:00000000
"StartWorkingOnly"=dword:00000000
"StartBell"=dword:00000001
"StartNotAfter"=""
"WindowState"=dword:00000000
"PriorityLevel"=dword:00000002
Programming to the Registry
If you feel comfortable programming to the registry, you'll certainly want
use the following functions.
Create a new subkey below HKEY_CURRENT_USER\Software\PACT Software\Timer\,
for example HKEY_CURRENT_USER\Software\PACT Software\Timer\MyTimerSet001\. In this key set
all values, or only those you need.
Here is a ready to use C source code
program to do just that. First you find the type definition, second the function to create
a new timer set, third, the function to write it to the registry, and fourth, a function
to execute PACTimer.exe to get the newly added subkey re-checked.
From your Visual Basic
program you can also access the registry by declaring the WINAPI functions RegCreateKeyEx,
RegSetValueEx, and RegCloseKey.
// --- Declarations for PACT Timer 98 -----
#define APPREGKEY ("Software\\PACT Software\\Timer")
typedef struct _TimerSet { // ts set for one timer
information
char SubKeyName[MAX_PATH];
char ExeDescription[101];
// max 100 (+ \0)
char ExePath[MAX_PATH];
char ExeParameters[MAX_PATH];
char ExeWorkDir[MAX_PATH];
char StartNextDate[20];
// 1998/03/12 10:20:00 max 20
(= 19 + \0)
char StartNotAfter[20];
UINT StartEverySec;
UINT StartSecAfterBoot;
DWORD StartActivated;
// default: 1 = yes
DWORD StartDeleteAfter;
// default: 0 = no, only deactivate
DWORD StartWorkingOnly;
// default: 0 = run every day
DWORD StartBell;
// default: 1 = yes, play sound
DWORD WindowState;
// 0-5
default: 0, normal
DWORD PriorityLevel;
// 0-3
default: 2, normal
} TIMERSET;
void main();
BOOL SetTimerSet(TIMERSET *pts);
void StartPACTimer();
// --- Example on how to init and save a new timer -----
// Copyright © 1993-1998 PACT on all examples and
materials. All rights reserved.
// The examples provided here are NOT guaranteed to work. No warranties, no liability, no
guaranty for fitness of code.
// --- main -----------------------------
void main()
{
TIMERSET ts;
SYSTEMTIME st;
lstrcpy(ts.SubKeyName, "MyTimerSet0001");
lstrcpy(pts->ExeDescription, "Test Timer");
lstrcpy(pts->ExePath, "Notepad.exe");
pts->ExeParameters[0] = 0;
pts->ExeWorkDir[0] = 0;
pts->StartEverySec = 0;
pts->StartSecAfterBoot = 0;
pts->StartActivated = 1;
// yes
pts->StartDeleteAfter = 0;
// no
pts->StartWorkingOnly = 0;
// no
pts->StartBell = 1; //
yes
pts->StartNotAfter[0] = 0;
pts->WindowState = 0; //
0-5 default: 0
pts->PriorityLevel = 2; //
0-3 default: 2
GetLocalTime(&st);
st.wMinute += 5;
if(st.wMinute > 59)
{
st.wMinute -= 60;
st.wHour++; // and so on...
}
wsprintf(ts.StartNextDate, "%04i/%02i/%02i %02i:%02i:%02i",
st.wYear, st.wMonth, st.wDay, st.wHour, st.wMinute, st.wSecond);
SetTimerSet(&ts); // save to reg
StartPACTimer(); // restart Timer
return;
}
// --- save timer to registry -------------------
BOOL SetTimerSet(TIMERSET *pts)
{
HKEY hkNewKey;
char szFullKeyName[MAX_PATH];
DWORD dwDummy;
DWORD dwReturn;
lstrcpy(szFullKeyName, APPREGKEY);
lstrcat(szFullKeyName, "\\");
lstrcat(szFullKeyName, pts->SubKeyName);
// Open/Create Key for write access
dwReturn = RegCreateKeyEx(HKEY_CURRENT_USER, szFullKeyName, 0, 0,
REG_OPTION_NON_VOLATILE, KEY_ALL_ACCESS, NULL, &hkNewKey, &dwDummy);
if(ERROR_SUCCESS != dwReturn)
{
SetLastError(dwReturn);
myErr("Error writing to registry! Can not
open application key.");
return FALSE;
}
// Set SZs
RegSetValueEx(hkNewKey, "ExeDescription", 0, REG_SZ, (CONST
BYTE *) pts->ExeDescription, lstrlen(pts->ExeDescription) + 1);
RegSetValueEx(hkNewKey, "ExePath", 0, REG_SZ, (CONST BYTE *)
pts->ExePath, lstrlen(pts->ExePath) + 1);
RegSetValueEx(hkNewKey, "ExeParameters", 0, REG_SZ, (CONST
BYTE *) pts->ExeParameters, lstrlen(pts->ExeParameters) + 1);
RegSetValueEx(hkNewKey, "ExeWorkDir", 0, REG_SZ, (CONST BYTE
*) pts->ExeWorkDir, lstrlen(pts->ExeWorkDir) + 1);
RegSetValueEx(hkNewKey, "StartNextDate", 0, REG_SZ, (CONST
BYTE *) pts->StartNextDate, lstrlen(pts->StartNextDate) + 1);
RegSetValueEx(hkNewKey, "StartNotAfter", 0, REG_SZ, (CONST
BYTE *) pts->StartNotAfter, lstrlen(pts->StartNotAfter) + 1);
// Set DWORDs
RegSetValueEx(hkNewKey, "StartEverySec", 0, REG_DWORD, (CONST
BYTE *) &pts->StartEverySec, sizeof(DWORD));
RegSetValueEx(hkNewKey, "StartSecAfterBoot", 0, REG_DWORD,
(CONST BYTE *) &pts->StartSecAfterBoot, sizeof(DWORD));
RegSetValueEx(hkNewKey, "StartActivated", 0, REG_DWORD,
(CONST BYTE *) &pts->StartActivated, sizeof(DWORD));
RegSetValueEx(hkNewKey, "StartDeleteAfter", 0, REG_DWORD,
(CONST BYTE *) &pts->StartDeleteAfter, sizeof(DWORD));
RegSetValueEx(hkNewKey, "StartWorkingOnly", 0, REG_DWORD,
(CONST BYTE *) &pts->StartWorkingOnly, sizeof(DWORD));
RegSetValueEx(hkNewKey, "StartBell", 0, REG_DWORD, (CONST
BYTE *) &pts->StartBell, sizeof(DWORD));
RegSetValueEx(hkNewKey, "WindowState", 0, REG_DWORD, (CONST
BYTE *) &pts->WindowState, sizeof(DWORD));
RegSetValueEx(hkNewKey, "PriorityLevel", 0, REG_DWORD, (CONST
BYTE *) &pts->PriorityLevel, sizeof(DWORD));
RegCloseKey(hkNewKey);
return TRUE;
}
// --- Start PACTimer.exe -----------------
void StartPACTimer()
{
HKEY hKey;
DWORD dwDummy = MAX_PATH;
char pszInstallPath[MAX_PATH] = {0};
char pszProgPath[MAX_PATH] = {0};
char pszTemp[MAX_PATH] = {0};
GetTempPath(MAX_PATH, pszTemp);
// get install path from Registry
if(ERROR_SUCCESS != RegOpenKeyEx(HKEY_LOCAL_MACHINE, APPREGKEY, 0,
KEY_QUERY_VALUE, &hKey))
return;
if( ERROR_SUCCESS == RegQueryValueEx(hKey, "InstallPath",
NULL, NULL, (LPBYTE) pszInstallPath, &dwDummy) )
{
lstrcpy(pszProgPath, pszInstallPath);
lstrcat(pszProgPath,
"\\PACTimer.exe");
ShellExecute(NULL, NULL, pszProgPath, NULL,
pszTemp, SW_SHOWNORMAL);
}
RegCloseKey(hKey);
return;
}
// --------------------------------------------
Support
Should you have technical questions please contact support@12Ghosts.com. We are known to solve issues
within hours.
We appreciate your suggestions and any feedback!
Timer Homepage
FAQ
Frequently Asked Questions
Options
and Registry Values
|
12Ghosts |

|
BackGhosts
2ndBackup - airbag for your data
ProfileCopy - copy user profiles,
including the referenced files!
Save Layout - save desktop icon layout into a file!
Timer - application scheduler, once, recurring,
after logon, reminder, moonphase
Do It Now
JumpReg - create bookmarks/ shortcuts
to registry keys, ZD:
SetTextColor - change the
desktop icon text and background colors, also transparent!
ShellX
New! context menu extension for user defined commands in the right-click
menu of files and folders!
ShutDown - without
confirmation, with one click, hotkey, shortcut, run program before!
Make it Easy
DeskTOP Desktop simulator -
application launcher with amazing behavior...
Quick Menu - open system folders and tools quicker!
It's ShowTime! - date and time always on TOP!
WinBzzzz - size and
position windows per click
How to order a license online, per e-mail, fax or mail
@ E-mail
technical support, questions and suggestions
|
Articles |
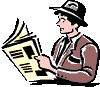
|
Code
Talk |
0101 0000 0100 0001 0100 0011 0101 0100
NT C VB PGP
XL
|
Personal |

|
|
Order License
@ E-Mail
PACT
|
|