By Lars Thomas Denstad <ign@styx.net>
|
Disclaimer: The contents of this document relect the author's views only. The author is not afiliated with Amiga, Inc in any way, except for being a registered user of the Amiga SDK. Also, please note: this article makes no attempt to cover all of the SDK; there is a lot of software in the package.
|
About the author
|
I was previously a die hard Amiga enthusiast. Recently my favorite desktop OS has become Linux (most distributions will do, currently running Linux Mandrake, Turbo Linux and Red Hat Linux) running Helix GNOME.
|
The SDK, as described in the sales ad
|
The details section in the product information at Amazon.Com promises the following:
|
- Amiga Foundation Layer
- Development tools for C, pJava and VP
- 100+ pages of documentation
|
Operating Environment
|
The Amiga SDK is sold as a software development kit, but also contains the Amiga OE runtime environment for Linux. This means that you will be able to run current and future Amiga applications on your Linux box, at speeds that are likely to be quite similar to a 'Native Next Generation Amiga OS'. This is great news for all of you out there that really like your current favorite OS, if it happens to be anything but AmigaOS.
|
A lot of people are asking about backward compability to the classic Amiga operating systems, and Amiga, Inc. is promising this through software emulation. While this is valuable, it's not what's really exciting about the design that's been chosen for the NG Amiga.
|
Amiga, Inc claims to have around 40 platforms ready to run VP. These platforms range from handhelds to servers. Given the broad range of platforms implementing some kind of Amiga NG runtime environment, my guess is that Amiga Classic is/will be one of them. This means in fact that not only do you have backwards compability from Amiga NG to Amiga Classic though software emulation, you will also have forward compability so you can run Amiga NG software on Amiga Classic.
|
It remains to be seen, however, how many of the media libraries are implemented on different platforms and in which ways the Amiga APIs will abstract the different facilities on a platform to see how instantaneously movable the code really is. The manual and supplied documentation files with the SDK does a fair job of describing the different abstraction layers, we'll look at that a little bit later.
|
The bottom line of what they are claiming is that you will be able to run the software created with the SDK on any platform that has an Amiga Operating Environment.
|
The package
|
The sporty-red package contains one CD and one manual. The CD contains about 28 MB of data. The manual is a 298 pages softcover book.
|
Installation: Red Hat Linux 6.1
|
I am currently running Linux Mandrake, and no matter how much they claim to require Red Hat 6.1, my first attempt at installing the SDK will be on this platform.
|
While firing up the installer was easy, and even though there was quite a lot of online messing around, the installation went smoothly. While it does install pretty easily, it does not run on Linux Mandrake 7.1.
|
Installation on Red Hat Linux 6.1 went as smoothly as on Linux Mandrake and the SDK comes up pretty easily. Don't be misguided by the manual, however. The following command lines are (to my knowledge) the correct ways of getting the environment up.
|
The intent shell: $ intent_shell
|
Intent Media: $ intent_media
|
The intent shell will give you a shell inside the current Linux terminal window, while intent_media will give you direct access to the graphical environment. Either one will work equally well, the Linux based intent shell will open the graphical window when needed.
|
The C-compiler
|
The C-compiler tool in the SDK is based on the GNU C Compiler. GCC is available for a bunch of different platforms. The Amiga SDK implements a new GCC backend, that is; instead of having GCC output Intel- or Alpha-binaries, it outputs VP code. The good thing about this approach is that you have a very thoroughly tested compiler that does the actual work; my guess is that the Amiga SDK-part mainly converts RTL (GCC's intermediate langauge) to VP.
|
I am very pleased with the choice of using GCC as the first compiler to do the Amiga SDK, not only is it widely and freely available, it is also a highly regarded product, and has always been a part of my favorite development-setups in the past.
|
Being how the Amiga SDK claims to have POSIX support, the natural first try-choice of porting effort had to be ddate , the Discordian Date command line utility.
|
The source is shipped with most Linux distributions, if you want to look at it yourself, you find it in the util_linux-package.
|
First tries at compiling gave some messages about missing include files, this was, however, not related to lacking POSIX compliance.
|
After about 15 lines of tweaking and some crippling of functionality, the app runs the way I usually start it, as you can see under the screenshots section.
|
The main reason I crippled some functionality was because of lacking random calls, this has similar functions in the Amiga API which they can be easily mapped to.
|
All in all, getting the app compiled and running was surprisingly easy.
|
VP
|
VP code did look kind of scary in the beginning, I must admit. For being 'assembly', however, it's pretty packed with features and easy to maneuver, even for me, having little or no exposure to assembly the last five years.
|
I wanted to write a little application that reads system clock, executes an application, reads the clock again, to see how long it took to execute.
|
After messing around in darkness for a couple of hours, I decided on the lib/microtime call, which has a simple interface.
|
VP allows you to work with the concept of both global and local variables. In this application, I wanted to store the start-time, the spawn-time and the end-time in global variables. The following codechunk 'declares' the variables I want:
|
structure int64 start_time int64 after_spawn int64 after_exec size TIMER_DATA_SZ
|
Note that this does not allocate the space to hold the variables, space for global variables are part of the tool-declaration:
|
tool 'app/timer',VP,TF_MAIN,1024,ARGV_DATA_SZ+TIMER_DATA_SZ
|
As you can see, I also want some global space to store the arguments passed to the application. VP happily allows expressions in a lot of places where you'd think it wouldn't. This is obviously a good thing.
|
As I wrote earlier, I decided to measure time with the tool lib/microtime . The code to call this tool looks as follows:
|
cpy.p NULL, p1 qcall lib/microtime,(p1 : i0)
|
When this code returns, the i0 register holds an indication of lapsed microseconds. If you do successive calls to this tool, you can calculate deltas. As I will be doing at least three calls, and I also want to store the result in variables, I decided to make a function of it:
|
time: ent p0 : - cpy.p NULL, p1 qcall lib/microtime,(p1 : i0) cpy.i i0, [p0] ret
|
This function takes one parameter in, as specified by the ent-line; ent p0 : - means that the first parameter should locally be assigned to the p0-register. You'll be happy to hear that during this call, the manipulation of p0 will not affect the 'global' p0, if you assign a NULL-pointer to p0 before the call, the p0 will still be NULL when the call returns. The dash behind the colon means that this function returns nothing. Now, to call it, you simply write:
|
gos time,(gp+start_time:-)
|
This call will put the current time into the global variable start_time . You use gp+variable_name to refer to global variables, gp being the globals pointer.
|
In the function time , surely you notice the use of hard brackets. In VP, they have the same function as the C-operator *, when used to follow a pointer. They can also be nested, so if you for instance have a pointer (p0 ) to an array of strings, printf "%s\n", [[p0]] will print the first.
|
VP also supports controlling flow through high-level calls like while ... endwhile , for ... next , etc., as well as if - and switch -statements.
|
The Amiga Foundation Layer
|
The Amiga Foundation Layer consists of a big range of tools. The term 'tool', when you're talking about the new Amiga OS, means the same as a dynamically loadable function. If you consider the previous section about VP, the call to lib/microtime is a typical call to a tool.
|
There is a distict difference between this, and the traditional 'shared library' (.dll, .so, etc). Tools are actually 'objects', that can be extended. People with some background from Object Oriented Programming, will feel right at home. The tools are also by definition both multi-threaded (thread-safe?) and re-entrant.
|
The Amiga SDK ships with a lot of different tools, ranging from encryption to GUI programming.
|
Screenshots
|
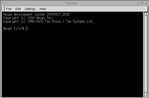 |
This is what it looks like when you do a $ intent_shell .
|
 |
UNIX-style $ ls . UNIX-users will be pleased to know that UNIX-toggles like "-alR" work as expected.
|
 |
The AMIGA ball bouncing happily on top of Red Hat Linux 6.1 with Helix GNOME. Please note that the artifacts in the screenshots are caused by JPEG-compression.
|
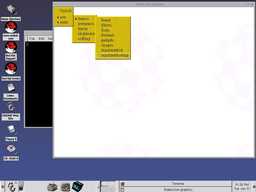 |
The root-menu. The contents of the menu are easily changed adding files to specified directories.
|
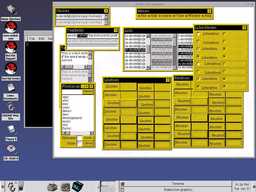 |
Running the gadget-menu. They look alright, and are pretty snappy, even on the machine that these pictures were taken on, which is a ~=200Mhz Pentium MMX with 128MB RM.
|
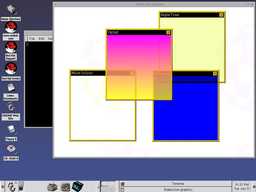 |
Different gradients alphablended. Surprisingly fast.
|
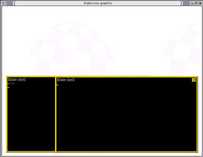 |
I've had some problems with eterm just not coming up correctly. This issue has been resolved with some help from our friends at Amiga Inc's support department.
|
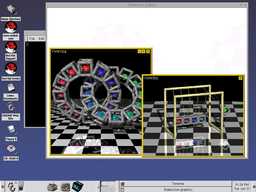 |
Image on the left is static, image on the right is animating.
|
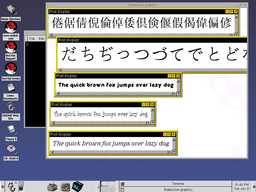 |
Demonstration of anti-aniased fonts.
|
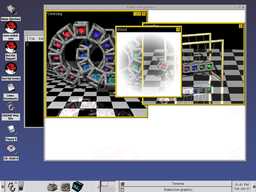 |
Nice effects, realtime demonstration of alpha-blending.
|
 |
Trying to cd to a directory that doesn't exist. Odd behaviour, but I'm sure it has some purpose. Update: The good folks at Amiga support de-mystifies: By allowing the user to cd to a directory that doesn't exist, you can later mkdir . . This is in many ways better than mkdir some_long_directory_name ; cd some_long_directory_name . Interesting.
|
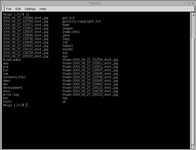 |
You can see how the screenshots I've been taking are being made available to the Amiga runtime environment.
|
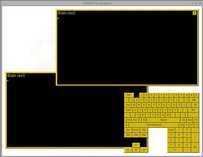 |
Two hung eterms and the soft keyboard, fully unfolded.
|
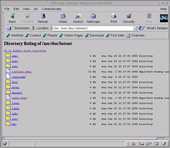 |
Linux Netscape browsing the intent online-documentation.
|
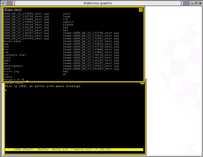 |
OK, with a little help from our friends at support, we have two eterms up, one just running the shell, one running the JOVE editor.
|
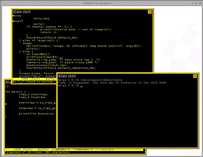 |
Ah, it seems like it's all starting to pay off. Downloaded the Linux source for ddate, and a few minutes, and about 15 tweaked lines later, it's running on the VP. The POSIX libraries are going to make it easy to port a lot of software to this platform.
|
|
Online support
|
The online support from Amiga, Inc is so far both impressive and a let-down. The development site is not yet online at the time of writing, and the shipped documentation leaves a lot to be desired. The people answering email at support@amiga.com are quick with so far a maximum 24h turnaround, and they've been able to resolve all my issues so far.
|
Downloads
|
Ok, ddate can be downloaded, but even though it runs nicely, I did just go ahead and remove some of the random-functionality as written before.
|
C-source and VP bytecode. Have fun. And celebrate Bureflux.
|
Conclusion
|
As a temporary conclusion, I must say, this is a fun package. Some shortcomings on the platform, documentation and especially (at the time of writing) web-resources for developers. But it looks very, very promising.
|
Most of the graphical applications can be described as 'snappy' even on the Pentium 200Mhz I'm running it on. The eterm is, however, quite slow, I'm sure this will improve shortly. I'll write some more when I get the SDK up and running on my AMD K7 750Mhz, and when I finish some simple tools for timing operations.
|
Amiga Inc should get http://www.amigadev.net online, and release some runtime-environments for different platforms, so we can get a taste on the portability of this thing.
|
One thing's for sure, if you want to be doing Next Gen Amiga development, the time to get started is now.
|
Related resources
|
Amiga Active is giving away five copies of the SDK in their latest issue.
|
Purchase the SDK online from Amiga, Inc.
|