
Programming for non-programmers
David Holden introduces a series showing beginners how to use
the powerful built-in Basic interpreter in your RISC OS machine.
Part 1 - Introduction
Once upon a time, many years ago, Acorn made the BBC computer. At the time this was a highly advanced machine, but it lacked one thing that makes modern computers 'user friendly' - it didn't have a WIMP front-end. The only way the user could give instructions to the computer was by a Command Line Interface, or CLI. Of course, at the time there was nothing unusual about this; all computers worked that way, including contemporary PCs running DOS.
In fact, the BBC computer didn't actually start up in a true CLI environment, it jumped straight into the Basic language and presented the user with a '>' prompt. This meant that the user could type Basic statements, but as any line which began with a '*' was passed directly to the CLI they would also have direct access the the Operating System or OS from the same '>' prompt.
When Acorn introduced its wonderful Archimedes computer, the ancestor of all current RISC OS machines, it did have a WIMP front-end of sorts. This was the infamous Arthur operating system. However, the WIMP was, to say the least, not very good so most users - the majority of whom had previously owned BBC micros - just configured their machines to start up in Basic with the familiar '>' prompt.
With the arrival of RISC OS 2 there was, at last, a really useful and powerful WIMP environment. This was a great step forward, making Acorn computers much easier to use, and for the first time it was possible for someone with no previous experience to run complex programs without ever having to see a '*' or '>' prompt or type any commands. Previously all users had been obliged to learn a little about the workings of the computer to operate it at all..
Now this is great if you just want to run programs. As a non-technical user all you have to learn is the 'basics' of operating the computer and, of course, the things specific to the program(s) you want to run. The disadvantage is that users are effectively isolated from the need to do anything other than make selections from windows and menus. There is no longer any requirement to know what is really happening behind the scenes. With the old BBC computers and the early Archimedes that Basic '>' prompt was almost impossible to ignore. With no WIMP, it was possible to write quite simple programs to perform quite complex tasks, as the user did not have to bother with WIMP protocols, windows, and all the other things that make programming in the Desktop so complicated. This activity was further encouraged by the magazines, all of which published 'listings' of programs that readers could type in themselves. (Incidentally, in the spirit of those days, you're encouraged to type in all listings that accompany this series, rather then simply copying and pasting them; it's the reason they're not stored elsewhere on the CD-ROM.)
In the last decade things have changed. There is less and less emphasis placed on programming, and where magazine articles do appear they are mainly aimed at improving the techniques of people who already understand the basics. Almost nothing is written for the complete novice, and, unlike the earlier generation of Acorn users who first came to computing in the days of the CLI, most modern users have never used a computer which did not have a WIMP interface and so have no idea of how to begin.
This series of articles will therefore begin at the beginning and assume no previous knowledge. I know it's going to be a bit boring for readers who already have some experience of programming, but if I begin anywhere but at the very start some people are going to be left behind.
But first...
What tools do you require to begin writing programs on a RISC OS computer? Well, later on you will need a few specialist items, but for the moment, absolutely nothing. Everything is already supplied with the computer. This tutorial is going to use the Basic language because the version supplied with your computer will serve perfectly well, and it's free.
Basic in an acronym for Beginners All-purpose Symbol Instruction Code. Don't be put of by the word 'beginners'. Basic was originally intended to be used as a teaching tool, but modern variants like the BBC Basic supplied with RISC OS, are extremely powerful and there's almost nothing you can't do with it.
I'm going to describe using Edit to write programs. Many people will prefer a more powerful editor like Zap or StrongEd. There's no reason why you can't use these if you prefer, my sole reason for using Edit is because everyone has it and so everyone will be able to duplicate exactly what I describe.
All programs are made up of two parts, code and data. Code is the actual instructions to the computer. Data is the material, of whatever type, that is displayed, manipulated, or otherwise operated upon by the code. No program, no matter how simple, can exist without both of these components. There must be code, because otherwise there would be nothing to do the work, and there must be some sort of data, because otherwise there would be nothing for the code to work upon.
In Basic, code is represented by keywords. These are always in upper case, and because Basic is designed to be written, read and used by humans, they are roughly analogous to English.
Data can be divided into two types, static and variable. As the name suggests, static data is fixed. For example, if your program needed to calculate the circumference of a circle from its radius you would need to multiply the radius by 3.1428. The radius would be variable data as its value would change for every different sized circle. The multiplier, 3.1428, is always the same, and so this would be fixed data. This type of fixed data would be referred to as a constant, meaning that it never changes, and is the same each time the program is run.
There are two other elements that may be present in Basic programs, operators and comments. Operators are mathematical symbols and, although they are not actually code, are an ingregient of the 'working' part of the program so can be regarded as part of the code. Comments are simply remarks included by the programmer to describe or clarify what the code is meant to do. They act as reminders, so that if you come back to a program that was written some time ago you will be able to understand what a particular section actually does. They may be unnecessary, and whether you use them or not is entirely up to you.�Comments are really not a part of the program. They are ignored completely when the program is run.
A very simple program
Let us begin by writing the simplest possible program. Run Edit, and, from the icon bar menu follow 'Create' to the sub-menu where you should select 'BASIC'. This will open an editing window.
Into this type:
PRINT "Hello World"
and press Return. Don't miss the double-quotes around "Hello World".
You now have a very simple program. Click Menu over the Edit window and follow 'Save' to the 'Save as' window and drag the file icon to a suitable filer window to save it. As this isn't anything particularly valuable a RAM disc might be the best place to avoid cluttering up your hard disc. As you selected 'BASIC' from the 'Create' sub-menu when you started this file it will be saved as a Basic program. When you have saved it, you just need to double-click on it to Run it. Assuming you haven't made any mistakes, a 'Command' window will open and you should see something like this:
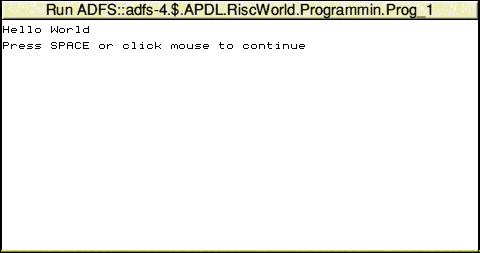
Now I accept that this isn't likely to impress your friends, but its main function was just to demonstrate how you Create, Save and Run a Basic program.
As you will gather, the keyword PRINT has nothing to do with printers. It will 'print' the data which follows to the computer screen. In fact, it's an extremely flexible and powerful command, and it can do more than just display simple text, as you will see later.
Variables
Although some data used by a program may be fixed, many things will alter, and so a program needs to be able to accommodate changing data. This type of data is stored in variables. There are different types of variable for different types of data. Every variable must be given a name, and is always referred to by that name. When a variable is 'created', Basic assigns an area of memory to accommodate the data. You don't need to know where that is, or how it is done, because you will not need to access that memory directly.
You can think of this as rather like a huge warehouse filled with racks. You never go into this warehouse; instead there's a man who does all the work for you. When you want to store something you give it to him and tell him a 'code name' to store it under. He vanishes into the warehouse, and somewhere a shelf is labelled with your code name and the item stored on that shelf. You don't know how or where the item is stored, you don't need to. When you want that item you just ask him to fetch it, calling it by the code name you'd given it.
By placing data in variables it can be altered as the program is running. Fixed data and constants cannot be changed.
In some programming languages variables must be declared before they are used, to allow the computer to set up memory space for them and know what sort of information is to be stored in them. In BBC Basic this is not necessary, they will be created 'on the fly' as a value is assigned to them.
In the BBC Basic as used with RISC OS there are a few constraints on the names that can be assigned to variables. I shall describe these later, but for the moment I would just suggest that you use only lower case letters. There are very good reasons for this, but one of the most important is that, as all kewords must be capital letters, it's a lot easier to 'read' a program and distinguish between keywords and variables if you make variables lower case.
Create a new Basic window in Edit and enter the following program.
first = 2
second = 3
sum = first + second
PRINT sum
The spaces are not important, I have just used them to make the code more clear.
Save this just as before and double-click on it to run it. You should see this:
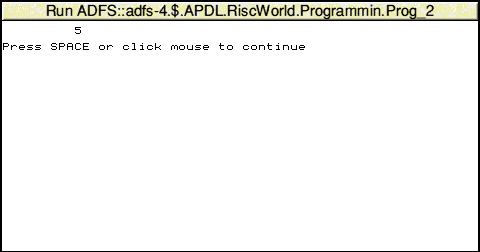
So, what's happening?
The first line of the program tells Basic to create a variable called first and assign the value '2' to it. The second line creates another variable, second and gives it the value '3'. The third line creates yet another variable sum, but this time, instead of assigning a fixed number to the variable, it gives it the value of first plus the value of second. When, in the last line, we use PRINT to display the value of sum it shows us that sum has a value of 5.
Some people have a problem understanding the use of '=' in this context, which is called an assignment. To aid understanding Basic has a keyword, LET , which does absolutely nothing, but which may help you to understand what's going on. The first line of the program could be wriiten as:
LET first = 2
which can make the meaning more obvious.
Interaction
Apart from trial programs like the ones above, which don't really perform any useful function, programs need to interact with the 'real' world. In fact, because programs are usually required to do something, they all tend to follow a pattern.
- Get data
- Process data
- Output results
The second program can perform the second two steps, that is, it 'processes' data (by adding together two numbers) and it 'outputs results' by PRINT ing the sum, but it's not much use because the two numbers it adds together, even though they are stored in variables, are the same every time. To make the program 'interact' we need a way of letting the user enter the numbers to be added together while the program is running.
The easiest way to do this is to use the Basic INPUT keyword. As a general rule, for reasons I shall explain later, this should not be used in a real, working, program, but it has the advantage that it's very simple and so we shall use it in these early stages.
You can either re-load the second program into Edit and alter it or begin a new Basic file. This time enter:
INPUT first
INPUT second
sum = first + second
PRINT sum
This time when you Run the program the Command Window will open, but all that will appear is '?'. This is a prompt and tells you that the program is expecting something. It's not very helpful, but we'll correct that later. Just type a number, say 7, and press Return. Another '?' will appear, so type another number, say 12, and press Return again. As soon as you do this the program will add the two numbers together and show you the result.
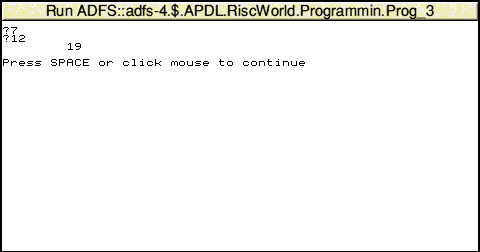
This time we've included the first step, getting data, so we have a program that can actually perform a 'real' task, even if it's somewhat trivial.
It's not very 'user friendly' though. Those question marks are a bit cryptic, so let's add something to tell the user what we expect. Change the program to the following:
INPUT "Enter first number " first
INPUT "Enter second number " second
sum = first + second
PRINT "The sum is " sum
When you run it now it will be a little more obvious what you have to do.
The text enclosed in double quotes after the INPUT keyword is displayed and the program will then wait for you to type the number. When you have done so it will assign the number to the specified variable. If there is no text after the keyword INPUT then, as in the earlier example, a simple '?' will be used.
With the INPUT keyword (as with PRINT ) if you want text to be displayed you must enclose it in double quotes.
Loopy programming
One of the most powerful aspects of computers is their ability to perform simple, repetitive tasks at great speed without getting bored. Our program so far is fine for adding two numbers together, but it only does it once. To get a program to perform a simple task like this many times we need a loop. To go back to our earlier definition of a program we can add a fourth item:
- Get data
- Process data
- Output results
- Go back to 1
As you can see, this structure on its own would make the program carry on forever.
To implement this in our program we need to add a new first line:
REPEAT
and a new last line:
UNTIL sum = 100
so the complete program becomes:
REPEAT
INPUT "Enter first number " first
INPUT "Enter second number " second
sum = first + second
PRINT "The sum is " sum
UNTIL sum = 100
You can probably guess what this will do even before you run the program. The keywords REPEAT and UNTIL are called a structure. This means that, although they are separate words, they are always used in combination. What we have created is called a REPEAT UNTIL loop. The nature of this type of loop is that it will repeat whatever is between the two keywords until the 'condition' after the keyword UNTIL is met. In this case, this means the program will just carry on asking for two numbers and adding them together until they add up to 100, then it will terminate.
In the last line of this program '=' is used in a different way. In the first three lines it is used to assign a value to a variable, in the last line it is used as a condition to compare two values.
Operators and Conditions
We now have a rather crude but functioning program that will add two numbers together. Obviously the computer is capable of more arithmetic functions than adding. The following characters are used for the four main functions:
- + for Add
- - for Subtract
- * for Multiply
- / for Divide
Basic can do more complex mathematical calculations, but we shall deal with those later.
We have seen how the '=' sign can be used to find out if two numbers are equal, and it is also possible to find out if one number is greater or smaller than another. These symbols are -
- > for Greater Than
- < for Less Than
- >= for Greater Than or Equal To
- <= for Less Than or Equal To
You should now be able to modify our simple program to add, subtract, multiply, or divide two numbers, and you should also be able to arrange for it to quit when the answer is less than or greater than a pre-determined number.
The END and Comments
So far our programs have simply stopped when they have come to their natural end. However, for reasons which will become apparent later, this is not the best way to terminate a program. The keyword END signifies the end of the program, and we shall use this in the future.
Earlier I mentioned comments in programs. In Basic this is done with the keyword REM (for REMark). If you begin a line with REM then the rest of the line will be ignored. It is a good idea to make the first line or two of a program a short reminder of what the program does.
A more useful program
We have seen how to write a program to do simple arithmetic, now we shall use the same techniques to calculate VAT. In fact, we will start out with two separate programs, one to add VAT to a price, and the second to deduct VAT from a VAT inclusive price. Here is the first:
REM Add VAT to a price
INPUT "Enter net price " price
cost = price * 1.175
PRINT "Price including VAT is " cost
END
and the second program:
REM Calculate net price from VAT inclusive price
INPUT "Enter total cost " cost
price = cost / 1.175
PRINT "Price without VAT is " price
END
There's nothing really new in either of these, and from what has gone before you should be able to see how they work.
It would be more useful if we could combine these two programs into one that could perform both tasks, namely, add or subtract VAT. To do this there must be some way of deciding which of the two options is to be used. This means choosing between two different options.
Choices, choices....
It is a fundamental requirement of programs that they must be able to choose between two or more options and act upon the choice made. The simplest method of making choices is the IF ... THEN ... ELSE structure. The specific representation of this structure is:
IF <condition> THEN <do something> ELSE <do something else>
The ELSE <do something else> part is optional.
We can use this system to tell the program whether we want it to add or subtract VAT by getting it to ask the user which function to perform and then acting differently depending on which choice the user makes.
PRINT "Do you want to -"
PRINT " 1. Add VAT to the net price"
PRINT " 2. Calculate net price from VAT inclusive price"
INPUT "Please enter 1 or 2" choice
As you should be able to work out from the earlier examples, this fragment of the program, when run, would display the following text on screen:
Do you want to -
1. Add VAT to net price
2. Calculate net price from VAT inclusive price
Please enter 1 or 2
You should be able to recognise this as a simple menu. Pressing either the '1' or '2' key and then RETURN will give the variable 'choice' the value of 1 or 2. We can then get the user to enter the price, and use the value of the variable 'choice' to decide what to do with it.
IF choice=1 THEN result = price * 1.175 ELSE result = price / 1.175
PRINT result
So, putting it all together, the program would be -
REM Program to add or subtract VAT from a price
PRINT "Do you want to -"
PRINT " 1. Add VAT to the net price"
PRINT " 2. Calculate net price from VAT inclusive price"
INPUT "Please enter 1 or 2 " choice
INPUT "Enter price " price
IF choice=1 THEN result = price * 1.175 ELSE result = price / 1.175
PRINT result
This will work, but it has a fundamental fault, and it's one that is often made by inexperienced programmers.
You can see what would happen if the user chooses 1 or 2, but what would happen if they entered something else, say 5, or if they just pressed Return when asked to choose? The IF ... THEN ... ELSE structure used is not very discriminating. If the user chooses 1 then the program will add VAT, but if they enter anything else it will subtract VAT.
There are two possible courses of action we could take to correct this. One is to tell the user that they have made an invalid choice, for example:
IF choice = 1 THEN result = price * 1.175
IF choice = 2 THEN result = price / 1.175
IF choice > 2 THEN PRINT "You must enter 1 or 2"
This would work, but a better method would be to check that the choice made was valid, and if not, tell the user and ask them to choose again. We can do this using the REPEAT ... UNTIL structure:
REPEAT
INPUT "Please enter 1 or 2 " choice
UNTIL choice = 1 OR choice = 2
You will notice that we have introduced a new keyword, OR . In this context it means what you would expect, namely that to exit from the loop either choice must equal 1 OR choice must equal 2. If neither of these conditions are met, the program will go back to the start of the loop and ask again.
By using this structure we can be sure that, when the program exits from the loop, the variable choice must have a value of either 1 or 2, so our simple IF ... THEN ... ELSE structure will suffice.
So, the finished program becomes:
REM Program to add or subtract VAT from a price
PRINT "Do you want to -"
PRINT " 1. Add VAT to the net price"
PRINT " 2. Calculate net price from VAT inclusive price"
REPEAT
INPUT "Please enter 1 or 2 " choice
UNTIL choice = 1 OR choice = 2
INPUT "Enter price " price
IF choice=1 THEN result = price * 1.175 ELSE result = price / 1.175
PRINT result
END
This seems a good point to end our first lesson. In a very short time you should have begun to understand the most fundamental aspects of programming. Although the VAT calculator is very crude, it can perform all the basis functions set out earlier in this lesson, and, in addition, it can make a choice between different options. In fact, although there's a long way to go, once you have grasped these few fundamental principles you will be well on the way to learning to write more complex programs.
David Holden

|